Gitlab has changed their Gitab Runner registration process from version 15.10. Previously there was a simple one step registration process where it was possible to register a runner by simply running gitlab-runner register command on the server/entity where the runner was installed. The old procedure was using a single registration token which was linked to the project and was used by all the runners registered for this one project.
The registration process now have changed from one step to two steps and each runner now will have to obtain their own unique authentication token.
Step 1: A new project runner will typically be created manually on the gitlab.com interface. ( scroll down for automation ).
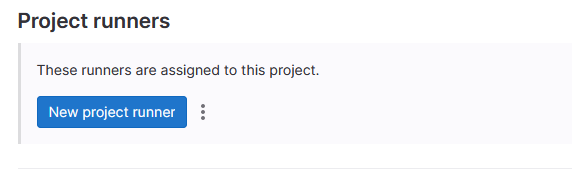
Many of the options previously set from gitlab-runner register command as a parameter, now will be set here instead. Such as run-untagged, locked, tag-list, etc.
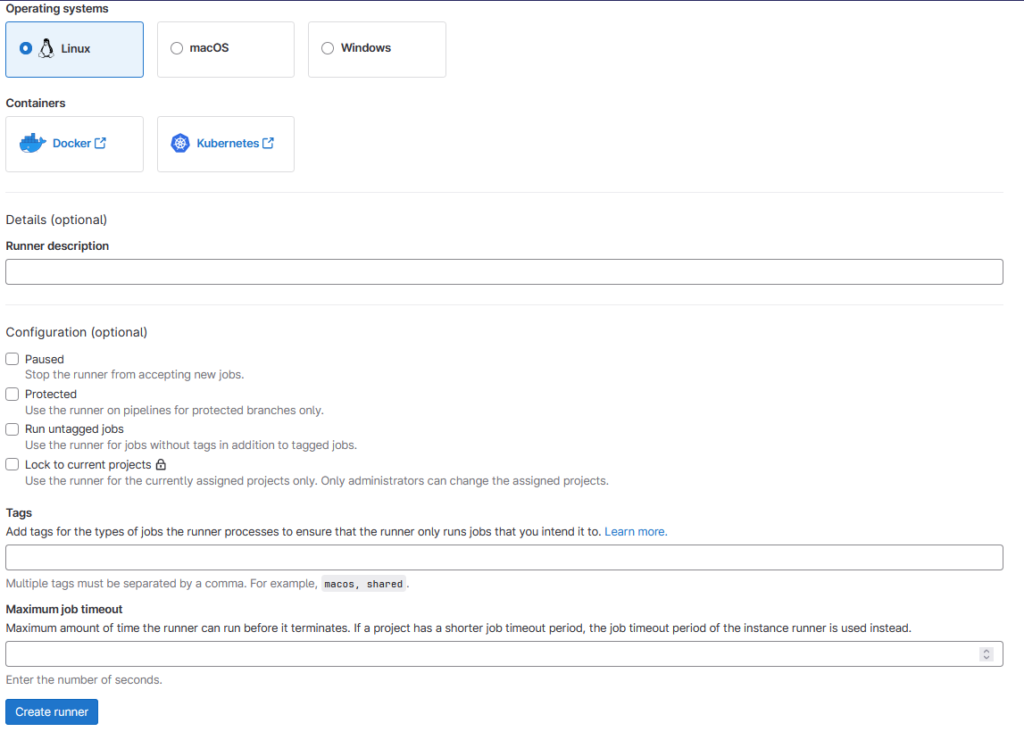
This new project runner will generate a unique authentication token.
Step 2: Run the gitlab-runner register command using this unique authentication token to link this runner with the project.
Needless to say that this breaks any kind of automation that has been created previously for runner registration so this would cause multiple issues for any customer using automatic registration.
After browsing some forums I found out that a new project runner can be created automatically using gitlab’s graphQL API. There is a mutation called RunnerCreate which does this part automatically. So running this call will return the authentication token, which then can be used to register the runner. I used the following call to get an authentication token:
mutation {
runnerCreate(
input: {projectId: "gid://gitlab/Project/00000000", runnerType: PROJECT_TYPE, tagList: "yourtag"}
) {
errors
runner {
ephemeralAuthenticationToken
}
}
}
Replace the 00000000 with your project id and it should create a new project runner and return the authentication token.
I have also written a bash script that would automate the entire process end to end. The script should be running on the server/entity where the runner is installed.
#!/usr/bin/bash
export PRIVATE_TOKEN="Replace with your Personal Access Tokens"
# Go to Settings -> General and copy the numeric value from "Project ID".
export PROJECT_ID="Replace with your Project ID"
export TAGLIST="yourtag"
export RUN_UNTAGGED="true"
export LOCKED="true"
# Change this is to your own hosted gitlab URL if you use gitlab.com leave the value set.
export GITLAB_URL="https://gitlab.com"
export TOKEN=$(curl "$GITLAB_URL/api/graphql" --header "Authorization: Bearer $PRIVATE_TOKEN" --header "Content-Type: application/json" --request POST --data-binary '{"query": "mutation { runnerCreate( input: {projectId: \"gid://gitlab/Project/'$PROJECT_ID'\", runnerType: PROJECT_TYPE, tagList: \"'$TAGLIST'\", runUntagged: '$RUN_UNTAGGED', locked: '$LOCKED'} ) { errors runner { ephemeralAuthenticationToken } } }"}' |jq '.data.runnerCreate.runner.ephemeralAuthenticationToken' | tr -d '"')
sudo gitlab-runner register --non-interactive --url $GITLAB_URL --token $TOKEN --executor shell
This script is also available on github.